@svelte-put/clickoutside
event for clicking outside node
Installation
terminal
npm install --save-dev @svelte-put/clickoutside
Quick Start
<script lang="ts">
import { clickoutside } from '@svelte-put/clickoutside';
function doSomething(e: CustomEvent<MouseEvent>) {
console.log(e.target);
}
</script>
<div use:clickoutside on:clickoutside={doSomething} />
Advanced Usage & Customization
Feature Demo
usage demo source. expand to see
<script lang="ts">
import { clickoutside } from '@svelte-put/clickoutside';
import { quintOut } from 'svelte/easing';
import { fly } from 'svelte/transition';
let enabled = true;
let parent: Element;
let click = 0;
let cls = '';
export { cls as class };
function onClickOutside() {
click++;
}
function toggleEnabled() {
enabled = !enabled;
}
</script>
<fieldset
class="border-4 border-blue-500 bg-violet-200 p-10 text-dark marker:text-dark prose-strong:text-dark {cls}"
bind:this={parent}
>
<legend class="font-bold text-blue-500">Limit</legend>
<div
class="grid border-2 border-gray-500 bg-gray-200 p-6"
use:clickoutside={{ enabled, limit: { parent } }}
on:clickoutside={onClickOutside}
>
<p>
<code>use:clickoutside</code> is registered for this <strong>gray box</strong>. Try these:
</p>
<ol>
<li>
Click within this <strong>gray</strong> zone => <strong>won't </strong> trigger
<code>on:clickoutside</code>
</li>
<li>
Click on the <strong>violet</strong> zone => <strong>will</strong> trigger
<code>on:clickoutside</code>
</li>
<li>
Click outside the <strong>blue</strong> limit => <strong>won't</strong> trigger
<code>on:clickoutside</code>
</li>
<li>Enable/disable <code>use:clickoutside</code> with button below, then try (2) again</li>
</ol>
</div>
<div class="flex items-center justify-between">
<p class="">
<code>on:clickoutside</code> counter:
{#key click}
<strong in:fly={{ y: 8, duration: 250, easing: quintOut }} class="inline-block"
>{click}</strong
>
{/key}
</p>
<button class="bg-blue-500 px-2 py-1 text-white" on:click|stopPropagation={toggleEnabled}>
{#if enabled}
Disable
{:else}
Enable
{/if}
</button>
</div>
</fieldset>
<svg use:clickoutside>
<g use:clickoutside></g>
<text use:clickoutside></text>
</svg>
clickoutside
Zone
Limit the As seen in the demo above, the limit.parent
option can be set to limit the zone
that will trigger on:clickoutside
. By default, there is no limit, and the event
listener is attached to document
.
setting limit.parent
<div bind:this={parentNode}>
<div use:clickoutside={{ limit: { parent: parentNode } }}>...</div>
</div>
Event Type Customization
By default, clickoutside
is based on the click
event. You can
customize this with the event
option.
overriding event type
<div use:clickoutside={{ event: 'mousedown' }}>...</div>
AddEventListenerOptions
Additional options can be passed to addEventListener should it be necessary.
providing AddEventListenerOptions
<div use:clickoutside={{ options: { passive: true } }}>...</div>
<div use:clickoutside={{ options: true }}>...</div>
clickoutside
Zone
Excluding Other Events in the In the
initial demo under the "Advanced Usage"
section, notice the stopPropagation
modifier was added to the click event. Without this, the button would also trigger an
on:clickoutside
event.
excluding events
<button on:click|stopPropagation>...</button>
Be aware to reflect the customization made to the event listener as mentioned in the last two sections. For example:
mousedown & capture
<div use:clickoutside={{ options: { capture: true }, event: 'mousedown' }}>...</div>
<button on:mousedown|stopPropagation|capture>...</button>
Another typical use case for this is shown below, where the same toggle callback is registered
for both on:clickoutside
and another click event.
|stopPropagation example
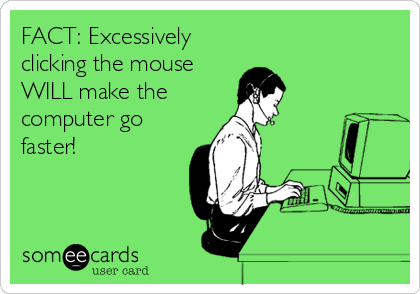
Happy clicking outside! 👨💻