@svelte-put/copy
action & utilities to copy text to clipboard
Installation
terminal
npm install --save-dev @svelte-put/copy
Usage
By default, copy
will register a click
1
event listener on the same node 2 it is used on. The triggered
listener will then copy the innerText
3 of the node
to the default clipboard.
default, no parameters
<script lang="ts">
import { copy, type CopyDetail } from '@svelte-put/copy';
import { fade } from 'svelte/transition';
let copied = '';
function handleCopied(e: CustomEvent<CopyDetail>) {
copied = e.detail.text;
}
</script>
<div class="not-prose grid grid-cols-[1fr,auto,1fr] items-center gap-2">
<button
class="bg-green-500 p-2 text-white active:scale-95"
type="button"
use:copy
on:copied={handleCopied}
>
Click <span class="text-blue-500">to copy this</span>
</button>
<div>-></div>
<div class="grid place-items-center self-stretch bg-blue-200 text-black">
{#if copied}
<p in:fade={{ duration: 200 }}>
{copied}
</p>
{/if}
</div>
</div>
The following sections show how 1, 2, 3 can be customized.
1 Customizing the Event Types
Pass one or more event types to the event
parameter.
specifying events
<script>
import { copy } from '@svelte-put/copy';
</script>
<button type="button" use:copy={{ event: 'mousedown' }}>...</button>
<button type="button" use:copy={{ event: ['pointerenter', 'pointerleave'] }}>...</button>
2 Customizing the Trigger
The button seen in all code blocks on this site is powered by this very action.
A typical use case is clicking on a node to copy the text of some other node.
custom trigger
3 Customizing How Text is Copied
The text
parameter can receive a string or a sync/async function that returns a
string, which if provided will be used for copying instead of the default
innerText
of the node.
Literal
custom text - literal
<button use:copy={{ text: 'some text from somewhere' }}>...</button>
Callback
Here, text
is a callback with the input containing information about the
forwarded event, reference to node
(on which action is used), and the
trigger
(to which event is attached).
custom text - callback
copy
event
Simulating the If synthetic
is set to true
, a "fake" copy
event will
be dispatched alongside copied
, should that be of any use.
Note that since this synthetic copy
event is not "real", and operations on
clipboardData
will have no effect on the actual copied text.
synthetic copy event
copyToClipboard
helper
Using the Behind the scene, copy
uses a copyToClipboard
helper. (You can see
its
source code here ).
You may skip the action and use this utility to build your own custom solution that fits your need.
copyToClipboard
import { copyToClipboard } from '@svelte-put/copy';
export function copy(text: string) {
copyToClipboard(text);
}
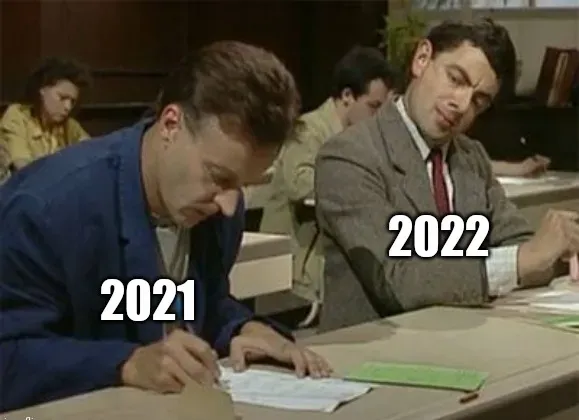
Happy copying! 👨💻